This sketch demonstrates a particle system with an object pool with automatic object cleaning and placement. The advantage of this approach over the confetti party demo is that no matter how long this demo runs there will be no performance degradation.
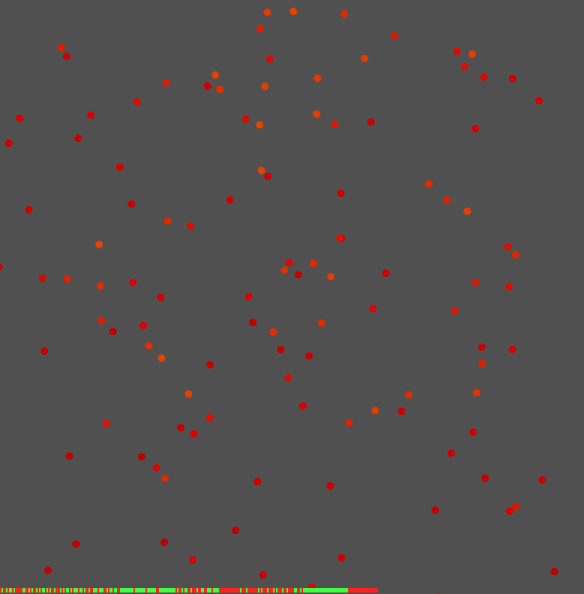
//Particle pool size of 256
let particlePool = new Array(256);
class Particle {
constructor(loc, vel) {
this.location = loc;
this.velocity = vel;
this.accleration = createVector(0,0.1);
this.colour = createVector(255,255,255);
}
update(){
let colourMin = createVector(-0.5,random(-1,-6),random(-2,-20));
this.velocity.add(this.accleration);
this.location.add(this.velocity);
this.colour.add(colourMin);
fill(this.colour.x,this.colour.y,this.colour.z);
stroke(color(0,0,0,0))
ellipse(this.location.x,this.location.y, 5, 5);
}
}
function newParticle(loc, vel){
let index = 0;
while((particlePool[index]!=undefined)&&(index<255)){
index++;
}
particlePool[index] = new Particle(loc, vel);
}
function cleanParticle(){
for(let i = 0;i<particlePool.length;i++){
if(particlePool[i] instanceof Particle){
if(particlePool[i].location.y>400){
particlePool[i] = undefined;
}
}
}
}
function updateParticles(){
for(let i = 0;i<particlePool.length;i++){
if(particlePool[i] instanceof Particle){
particlePool[i].update();
}
}
}
function mousePressed(){
for(let i = 0;i<25;i++){
newParticle(createVector(mouseX,mouseY),createVector((Math.random() * 5 - 2.5),(Math.random() * 5 - 5)));
}
}
function setup() {
createCanvas(400, 400);
}
function draw() {
background(80);
updateParticles();
cleanParticle();
for(let j = 0;j<particlePool.length;j++){
if(particlePool[j]==undefined){
fill(255,32,32);
}else{
fill(64,255,64);
}
rect(j,394,1,3);
}
}
Code language: JavaScript (javascript)